Creating my first python AWS script for automation
- Rebecca Boardman
- Apr 2, 2021
- 3 min read
So I’ll be the first to admit my python skills definitely still have a long way to go but this week as I was sat there reading through a documented process a thought came to me- surely this could be automated into a python script! So with my very limited knowledge of python let’s try it!
PreRequisite
Have AWS SSO (single sign on) profiles set up
What does the script do?
Well if your working In AWS and dealing with lots of different accounts and using AWS systems manager session manager to allow you to RDP onto an EC2 instance then your probably use to this command
aws ssm start-session --target <instance-id> --document-name AWS-StartPortForwardingSession --parameters "localPortNumber=55678,portNumber=3389" --profile ssoprofilename
If your dealing with lots of different profiles/accounts then getting the instanceID can be a bit of a laborious task. Not to mention if for whatever reason the instance got terminated and a new one was spun up it could have a different instanceID.
Where did I start?
Well, having only most recently started looking at python Ive been aware of the use of importing library's one of those being boto3 (AWS SDK).
I know it was possible to be able to describe your EC2 instances and return back an instance ID, this would be perfect for what I need. I just need to add the relevant filters (in this case name) and I was good to go. Boto3 also has some pretty good documentation online for all the possible commands you can run.
So I created the below script which returned my instances associated with my account
import boto3
boto3.setup_default_session(profile_name='Becky_TestAcc')
ec2 = boto3.resource('ec2')
for instance in ec2.instances.all():
print (instance.id)
Next I wanted to filter based on only returning EC2 instances where they were actually running and looking for a specific name. In my case the EC2 instances that I wish to connect to are all called *JmpSvr. "*" is a wildcard so it will returning anything which ends with JmpSvr. This time I used the boto 3 ec2 client describe_instances as it would allow me to filter. You can find the documentation on this here
import boto3
boto3.setup_default_session(profile_name='Becky_TestAcc')
clientec2 = boto3.client('ec2')
resp = clientec2.describe_instances(Filters=[{'Name': 'tag:Name', 'Values': ['*JmpSvr']},
{'Name': 'instance-state-name','Values': ['running']},
])
for reservation in resp ['Reservations']:
for instance in reservation['Instances']:
id = ("{}".format(instance['InstanceId']))
print (id)
The finished result
So now I've returned my instanceID I wanted to be able to execute a command to start the SSM session. So I imported the OS library to allow me to do that. I also added the ability to input your profilename your using for SSO rather than have a hardcoded value. Here is the finished result:
import boto3
import os
awsprofile = input ('Enter Name of SSO Profile:')
print (awsprofile)
boto3.setup_default_session(profile_name=awsprofile)
clientec2 = boto3.client('ec2')
clientssm = boto3.client('ssm')
resp = clientec2.describe_instances(Filters=[{'Name': 'tag:Name', 'Values': ['*JmpSvr']},
{'Name': 'instance-state-name','Values': ['running']},
])
for reservation in resp ['Reservations']:
for instance in reservation['Instances']:
id = ("{}".format(instance['InstanceId']))
print (id)
os.system('aws ssm start-session --target {} --document-name AWS-StartPortForwardingSession --parameters "localPortNumber=55679,portNumber=3389" --profile {} --region eu-west-2'.format(id, awsprofile))
Then all you need to do is save this script- I saved it as "ssmstartsession.py" within my python scripts and execute it like below:
python ssmsstartsession.py
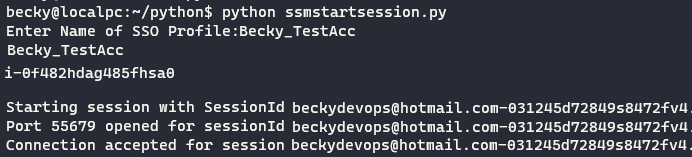
and as per above you can see it has successfully started a session and opened local port 55679. Allowing me to RDP on as below:
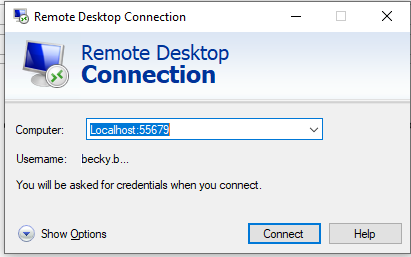
So there you have it! My first python script created from a thought in my head. It might seem really simple but I love having an idea and then be able to bring that idea to life, I'm looking forward to creating more scripts as the most I learn the better I'll be!
Comments